100 Days of Code Challenge: Python
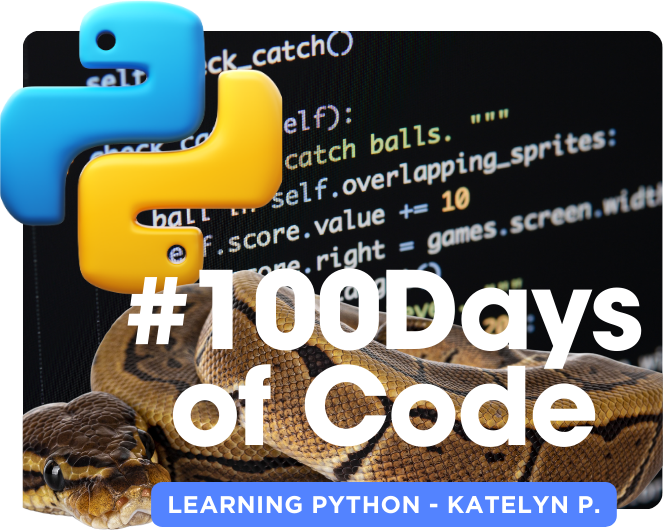
Welcome to the beginning of an exhilarating coding adventure! Over the next 100 days, I’m diving deep into the world of Python programming—a journey filled with learning, growth, and endless possibilities. This living blog is your front-row seat to witness the highs, the lows, and the incredible transformations that happen when dedication meets determination. Join me as we unravel the mysteries of Python, tackle challenges head-on, and embark on a transformative 100-day coding journey.
Day 1: Printing
On Day 1 of this Python adventure, we start with the basics: printing. It might seem simple, but this humble command lays the foundation for everything we’ll build in the days to come. The print()
function is our gateway into the world of Python output. Today, we explore its power, experimenting with text, numbers, and maybe even a few surprises. Stay tuned as we take our first step into the vast universe of Python programming!
The print
command in Python is used to display information. Here’s a short example:
# Define a variable
greeting = "Hello, Python!"
# Use the print command to display the content of the variable
print(greeting)
When you run this Python script, it will output:
Hello, Python!
The print
statement is a simple and essential tool for displaying information, whether it’s variables, strings, or the result of expressions, in the console or terminal.
Using Quotes
In Python, quotes are used to denote strings. Here are some cliff notes about single quotes, double quotes, and triple quotes:
- Single Quotes (
'
)- Used to represent a string in Python.
- Strings created with single quotes and double quotes are functionally equivalent.
- Example:
my_string = 'Hello, World!'
- Double Quotes (
"
)- Also used to represent strings.
- Similar to single quotes, interchangeable in most cases.
- Example:
my_string = "Hello, World!"
- Triple Quotes (
'''
or"""
)- Used for multiline strings or strings that span across multiple lines.
- Allow you to include line breaks within the string without using escape characters like
\n
. - Example:
my_multiline_string = ''' This is a multiline string '''
The choice between using single, double, or triple quotes often comes down to personal preference or specific requirements for string formatting or content.
Let’s dive into examples for each type of quote and provide a beginner-friendly definition of a string.
Single Quotes ('
)
# Example of a string using single quotes
my_string_single = 'Hello, World!'
print(my_string_single)
Double Quotes ("
)
# Example of a string using double quotes
my_string_double = "Hello, World!"
print(my_string_double)
Triple Quotes ('''
or """
)
# Example of a multiline string using triple quotes
my_multiline_string = '''
This is a
multiline
string
'''
print(my_multiline_string)
String
In programming, a string is a sequence of characters. It could be letters, numbers, symbols, or spaces enclosed within single quotes, double quotes, or triple quotes. Strings are used to represent text in computer programs.
For instance, in Python:
my_string = 'Hello, World!'
Here, 'Hello, World!'
is a string, indicated by the single quotes enclosing the text. Strings allow us to work with and manipulate textual data in our programs.
String Manipulation and Code Intelligence
Using a single print()
statement and \n
for new lines is a game-changer in Python. With this combo, we can elegantly format output across multiple lines, adding clarity and structure to our code. Today, we’ll harness the power of this simple technique to create tidy and readable displays within our scripts.
Here’s an example using a single print()
statement and \n
for creating multiline output:
# Example of using a single print statement and \n for new lines
print("Welcome to Python!\n")
print("Let's explore the power of Python programming.\n")
print("With code, creativity knows no bounds.\n")
This code will generate the same multiline output:
Welcome to Python!
Let's explore the power of Python programming.
With code, creativity knows no bounds.
In this example, \n
is used within the string to create new lines, maintaining the multiline structure within a single print()
statement.
Concatonating Strings
In the world of Python, string concatenation is the art of merging strings together. Using the +
operator, we can seamlessly combine multiple strings into one, creating a cohesive and unified output. This simple yet powerful technique allows us to dynamically build complex messages or manipulate text data. Today, we’ll explore the elegance and flexibility of string concatenation, unlocking its potential to craft cohesive narratives or construct intricate data structures within our Python code.
Here’s an example demonstrating string concatenation in Python:
# Example of string concatenation
first_name = "John"
last_name = "Doe"
full_name = first_name + " " + last_name
print("Full Name:", full_name)
# Concatenating strings with additional text
message = "Hello, " + full_name + "! Welcome to our Python community."
print(message)
Output:
Full Name: John Doe Hello, John Doe! Welcome to our Python community.
In this example, string concatenation using the +
operator merges first_name
and last_name
variables into full_name
. Additionally, the message
variable demonstrates combining strings with additional text to create a personalized greeting.
Here is another example:
print ("Hello" + input(" What is your name?"))
Output:
What is your name?Katt
Hello Katt
Input Function and Length
In Python, the input()
function opens a gateway for user interaction, allowing us to receive input directly from the user. This function prompts the user to enter data, which we can then manipulate and analyze within our programs. Additionally, calculating the length of the input, achieved through the len()
function, provides insight into the amount of data entered. Let’s explore how these tools work together in a simple example:
# Using input() function and calculating length
user_input = input("Enter a sentence: ")
input_length = len(user_input)
print("You entered:", user_input)
print("Length of the input:", input_length)
Nested Functions
Nested functions in Python refer to the concept of using one function inside another function. In the example print(len(input("What is your name?")))
, there’s a nested structure involving three functions: print()
, len()
, and input()
.
Example:
print(len(input("What is your name?")))
Output:
What is your name?Katelyn
7
Let’s break it down:
input("What is your name?")
: This prompts the user to input their name.len()
: Thelen()
function calculates the length of the string entered by the user.print()
: Finally, theprint()
function displays the length of the input received from the user.
This one-liner effectively gathers the user’s input (their name in this case), immediately calculates the length of the input string, and then prints out that length in a single line.
In essence, this nested function structure allows for a concise execution of tasks in a single line of code. The output becomes the length of the string entered by the user in response to the prompt for their name.
Variables
In Python, variables act as containers to hold various types of data. They serve as placeholders, allowing us to store, manipulate, and access information within a program.
Example:
name1 = input("What is your name?")
print (name1)
Output:
name1 = input("What is your name?")
print (name1)
Here is another Example:
# Example of variables in Python
name = "Alice"
age = 30
In this example, name
and age
are variables. name
holds a string value "Alice"
, while age
contains the numeric value 30
. What’s remarkable about Python variables is their dynamic nature; they can store different types of data and their types can change based on the assigned value. This flexibility makes Python versatile and conducive to rapid development.
Str and Len Function
The str()
and len()
functions are two different functions in Python:
str()
Function: Thestr()
function is used to convert values into a string format. For instance, if you have a number and want to use it as part of a string, you can convert it usingstr()
. Example:str(10)
would convert the number10
into the string'10'
.len()
Function: Thelen()
function, on the other hand, is used to determine the length of a sequence (such as a string, list, tuple, etc.). It returns the number of items in the sequence. For example,len("Hello")
would return5
, as there are five characters in the string “Hello”.
When used together like str(len(some_sequence))
, it computes the length of some_sequence
using the len()
function and then converts that length value into a string using str()
. This can be helpful when you want to obtain the length of a sequence and represent it as a string, perhaps for displaying information or combining it with other strings.
Example:
name1 = input("What is your name?")
print(name1 + " has " + str(len(name1)) + " letters in their name.")
Output:
What is your name?Darma
Darma has 5 letters in their name.
Understanding how to declare and utilize variables is fundamental to programming in Python. They allow us to store and work with data efficiently, enabling the creation of complex and dynamic programs.
Understanding .lower()
Method
In Python, the .lower()
method is a powerful tool for string manipulation. It allows us to convert all characters in a string to lowercase. This method comes in handy when we want to standardize or compare strings without considering their cases.
Example:
# Using the .lower() method
message = "HELLO, World!"
lower_case_message = message.lower()
print(lower_case_message)
or
ask3 = input('Where do you live? ')
if ask3.lower() == 'indonesia':
print('Awesome')
This feature proves beneficial due to Python’s case sensitivity. In an if
statement, without using .lower()
, user input would need to precisely match the defined case to validate the if
condition. This behavior may not always yield the desired outcome, as it requires an exact case match, potentially causing issues with validation.
Understanding Python’s Equality Operator: The == Sign
In Python, the ==
is an equality operator used for comparison. It checks if the values on the left and right sides of the operator are equal. If they are equal, it returns True
; otherwise, it returns False
.
Example:
ask3 = input('Where do you live? ')
if ask3.lower() == 'indonesia':
print('Awesome')
The ==
operator is used to compare values, and it’s important to differentiate it from the assignment operator =
. The =
operator is used to assign values to variables, while ==
is used for comparison.
Day 2: Data types and String Manipulation
In Python, understanding data types is fundamental to effectively working with information within a program. Let’s dive into the basic data types Python offers:
- Integers (
int
): Integers represent whole numbers without decimals. For example,5
and-10
are integers in Python. - Floating-Point Numbers (
float
): Floating-point numbers are used to represent real numbers and include a decimal point. For instance,3.14
or-0.5
are floating-point numbers. - Strings (
str
): Strings are sequences of characters enclosed in single quotes ('text'
) or double quotes ("text"
). They represent textual data. For example,"Hello, Python!"
is a string. - Booleans (
bool
): Booleans have only two possible values:True
orFalse
. They are often used in conditions and comparisons. - Lists (
list
): Lists are ordered collections of items, which can be of different data types and are enclosed in square brackets ([ ]
). For instance,[1, 2, 3]
or['apple', 'banana', 'cherry']
are lists. - Tuples (
tuple
): Tuples are similar to lists but are enclosed in parentheses (( )
). Unlike lists, tuples are immutable, meaning their elements cannot be changed after creation. For example,(1, 2, 3)
is a tuple. - Dictionaries (
dict
): Dictionaries are collections of key-value pairs, enclosed in curly braces ({}
). They allow us to store data in a structured format where each value is accessed by its associated key. For instance,{'name': 'Alice', 'age': 30}
is a dictionary.
Understanding these data types helps in manipulating and organizing data efficiently within Python programs. Python’s flexibility with different data types makes it a versatile language for various applications.
As we delve deeper into Python programming, exploring these data types will be essential for writing effective and robust code.